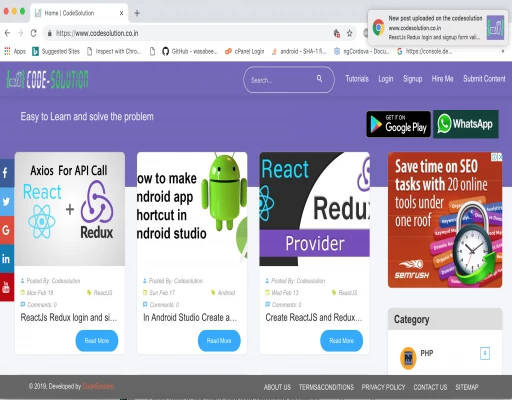
Firebase web push notification (firebase cloud messaging) with click event on notification in Javascript and php
Firebase
Web
Push Notification
notification
Javascript
php
- By Code solution
- Jan 20th, 2021
- 0 comments
- 2
The FCM JavaScript API lets you receive notification messages in web apps running in browsers that support the Push API. This includes the browser versions listed in this support matrix.
Firebase Cloud Messaging (FCM) is a cross-platform messaging solution that lets you reliably deliver messages at no cost.
FCM server has two components for sending and receiving messages which are:
- app server
- FCM servers
It is the job of the app server to send the message to the FCM server which then sends the message to the client device.
Folder Structure :
firebase-messaging-sw.js
php
action.php
DbConnect.php
index.php
manifest.json
send.php
img
image.png
image1.png
- Create a project on firebase console link
- After createing project click "+ Add app" button then select web platform and copy then code
- After then copy code paste index.php like that
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<title>Web notification</title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<script src="https://www.gstatic.com/firebasejs/5.8.3/firebase.js"></script>
<script>
// Initialize Firebase
/*Update this config*/
var config = {
apiKey: "<Enter your apiKey>",
authDomain: "<Enter your authDomain>",
databaseURL: "<Enter yourdatabaseURL>",
projectId: "<Enter your projectId>",
storageBucket: "<Enter your storageBucket>",
messagingSenderId: "<Enter your messagingSenderId>"
};
firebase.initializeApp(config);
</head>
<body>
</body>
</html>
- After then add code for Retrieve Firebase Messaging object
messaging.requestPermission()
.then(function() {
console.log('Notification permission granted.');
// TODO(developer): Retrieve an Instance ID token for use with FCM.
if(isTokenSentToServer()) {
console.log('Token already saved.');
} else {
getRegToken();
}
})
.catch(function(err) {
console.log('Unable to get permission to notify.', err);
});
function getRegToken(argument) {
messaging.getToken()
.then(function(currentToken) {
if (currentToken) {
//saveToken(currentToken);
var token = currentToken;
var device_id = '<?php echo md5($_SERVER['HTTP_USER_AGENT']); ?>';
console.log(token, device_id);
saveToken(token, device_id);
} else {
console.log('No Instance ID token available. Request permission to generate one.');
//setTokenSentToServer(false);
}
})
.catch(function(err) {
console.log('An error occurred while retrieving token. ', err);
// setTokenSentToServer(false);
});
}
window.localStorage.setItem('sentToServer', sent ? 1 : 0);
}
return window.localStorage.getItem('sentToServer') == 1;
}
$.ajax({
url: 'server url',
method: 'post',
data: {'device_id':deviceid, 'token':currentToken}
}).done(function(result){
console.log(result);
})
}
messaging.onMessage(function(payload) {
console.log("Message received. ", payload);
var notificationTitle = payload.data.title;
const notificationOptions = {
body: payload.data.body,
icon: payload.data.icon,
image: payload.data.image,
click_action: "https://www.examples.com/"+ payload.data.url, // To handle notification click when notification is moved to notification tray
data: {
click_action: "https://www.examples.com/"+ payload.data.url
}
};
var notification = new Notification(notificationTitle,notificationOptions);
});
- After then open firebase-messaging-sw.js and add code
importScripts('https://www.gstatic.com/firebasejs/4.8.1/firebase-messaging.js');
/*Update this config*/
var config = {
apiKey: "<Enter your apiKey>",
authDomain: "<Enter your authDomain>",
databaseURL: "<Enter yourdatabaseURL>",
projectId: "<Enter your projectId>",
storageBucket: "<Enter your storageBucket>",
messagingSenderId: "<Enter your messagingSenderId>"
};
firebase.initializeApp(config);
messaging.setBackgroundMessageHandler(function(payload) {
console.log('[firebase-messaging-sw.js] Received background message ', payload);
// Customize notification here
const notificationTitle = payload.data.title;
const notificationOptions = {
body: payload.data.body,
icon: payload.data.icon,
image: payload.data.image,
click_action: "https://www.examples.com/"+ payload.data.url, // To handle notification click when notification is moved to notification tray
data: {
click_action: "https://www.examples.com/"+ payload.data.url
}
};
self.addEventListener('notificationclick', function(event) {
console.log(event.notification.data.click_action);
if (!event.action) {
// Was a normal notification click
console.log('Notification Click.');
self.clients.openWindow(event.notification.data.click_action, '_blank')
event.notification.close();
return;
}else{
event.notification.close();
}
});
notificationOptions);
});
// [END background_handler]
Note: firebase-messaging-sw.js file available on root folder
you can used above method then create will a firebase token on website then you are used this token to test web push notification.
Send notification using php
- Create DbConnect.php file and add code
class DbConnect {
private $host = 'localhost';
private $dbName = 'fcm-push';
private $user = 'root';
private $pass = '';
public function connect() {
try {
$conn = new PDO('mysql:host=' . $this->host . '; dbname=' . $this->dbName, $this->user, $this->pass);
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
return $conn;
} catch( PDOException $e) {
echo 'Database Error: ' . $e->getMessage();
}
}
}
?>
- After that create action.php file and add the code
if(isset($_POST['token'])) {
require 'DbConnect.php';
$db = new DbConnect;
$conn = $db->connect();
$cdate = date('Y-m-d');
$stmt = $conn->prepare('INSERT INTO tokens VALUES(null, :token, :cdate)');
$stmt->bindParam(':token', $_POST['token']);
$stmt->bindParam(':cdate', $cdate);
if($stmt->execute()) {
echo 'Token Saved..';
} else {
echo 'Failed to saved token..';
}
}
?>
- Then create send.php file and add the below code
define('SERVER_API_KEY', 'YOUR SERVER KEY');
require 'DbConnect.php';
$db = new DbConnect;
$conn = $db->connect();
$stmt = $conn->prepare('SELECT * FROM tokens');
$stmt->execute();
$tokens = $stmt->fetchAll(PDO::FETCH_ASSOC);
foreach ($tokens as $token) {
$registrationIds[] = $token['token'];
}
'Authorization: Key=' . SERVER_API_KEY,
'Content-Type: Application/json'
];
$msg = [
'title' => 'FCM Notification',
'body' => 'FCM Notification from codesolution',
'icon' => 'img/icon.png',
'image' => 'img/c.png',
];
$payload = [
'registration_ids' => $registrationIds,
'data' => $msg
];
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://fcm.googleapis.com/fcm/send",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => json_encode( $payload ),
CURLOPT_HTTPHEADER => $header
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
?>
You can easy to implement (FCM) web push notification follow this instruction.
Note: Firebase web notification work only (Hyper Text Transfer Protocol Secure) https:// not working (Hyper Text Transfer Protocol) http://
Thanks